CORS on the Drogon web framework
CORS, the problem that every web developer has faced at least once in their life. Drogon does not come with built-in middleware to handle CORS, but it is easy to implement it yourself.
Usually, you'll want to expose your APIs to the world. This code will allow any request to the /api
path to go through, and will respond to any OPTIONS
request with the appropriate CORS headers.
using namespace drogon;
app().registerPreRoutingAdvice([](const HttpRequestPtr &req,
FilterCallback &&stop,
FilterChainCallback &&pass)
{
// Let anything not starting with /api or not a preflight request through
if(!req->path().starts_with("/api") || req->method() != Options) {
pass();
return;
}
auto resp = HttpResponse::newHttpResponse();
resp->addHeader("Access-Control-Allow-Origin", "*");
resp->addHeader("Access-Control-Allow-Methods", "GET, POST, PUT, DELETE, OPTIONS");
// Add other CORS headers you need
stop(resp); // stops processing the request and sends the response
}
Depending on your use case you might want to try pre-handling adivce if you want to exclude static files from CORS handling. Alternatively, you can add your own hander to APIs individually, like this:
Task<HttpResponsePtr> MyController::myApi(HttpRequestPtr req)
{
if(req->method() == Options) {
auto resp = HttpResponse::newHttpResponse();
resp->addHeader("Access-Control-Allow-Origin", "*");
resp->addHeader("Access-Control-Allow-Methods", "GET, POST, PUT, DELETE, OPTIONS");
// Add other CORS headers you need
co_return resp;
}
// Your API code here
...
}
That's it. It is possible to use a filter for this. But I never find it specially fitting better then AOP. I only use filters when a few APIs have special authentication requirements. AOP is much suitable for these wide area concerns.
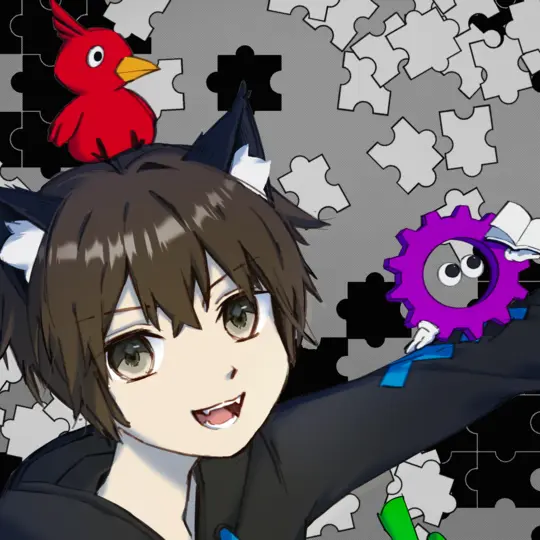
Martin Chang
Systems software, HPC, GPGPU and AI. I mostly write stupid C++ code. Sometimes does AI research. Chronic VRChat addict
I run TLGS, a major search engine on Gemini. Used by Buran by default.
- martin \at clehaxze.tw
- Matrix: @clehaxze:matrix.clehaxze.tw
- Jami: a72b62ac04a958ca57739247aa1ed4fe0d11d2df